NOTE: Make sure this procedure is done in the proper layer! AKA don't do it in the USR layer.
Step 1: Update the document service (Figure 1 below).
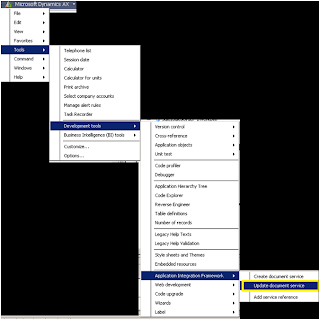
Figure 1 - Dynamics AX -> Tools -> Development Tools -> Application Object Framework -> 'Update Document Service'
Step 2: Select the service class name that will need to be updated (Figure 2 below). The class name will end with '…Service'. Any other class will give an error saying that the class is not a valid service class. Check the two supporting classes options in this feature.
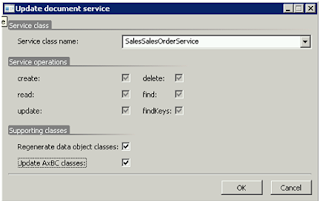
Figure 2 – Updating the Sales Order webservice
Step 3: There may be a number of errors in one of the web services classes (mine was the sales table header class). There seemed to be a problem generating the macros used in the code. I just added them to the class dec of that class and moved on.
Step 4: Regenerate the webservice
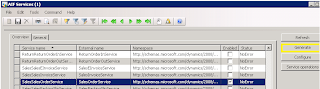
Figure 3 – Regenerate the Sales Order webservice
Step 5: Enable the Action Data Policy fields for the endpoints that are currently using this webservice. Since the data policies are governed on an Endpoint by Endpoint level, this is where these new fields will need to be turned off/on. This can be accessed by going to
-Basic -> Setup -> Application Integration Framework
-Select appropriate endpoint id
-Click 'Action policies' button
-Select appropriate Action policy
-Click 'Data policies' button.
-Enable new fields
Once these steps are complete, the web service's schema will allow for additional fields to be changed to allow these new fields to be integrated into the application.
NOTE: The underlying class may need to be deleted in order for things like string size to properly update in the Schema.
Enjoy!